Understanding wrapping and ordering in Flexbox
- CSS
In my last post I explained flex-direction. In this post I will be explaining flexbox-wrap
and order
.
flex-wrap
By default the flex items will shrink if there is not enough room in container instead of moving to the next row. This happens because flex-wrap
is set to nowrap
by default and it stops flex items from wrapping.
To understand how flex-wrap
works, I am going to use the code below:
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
</div>
.container {
display: flex;
border: 10px solid #000000;
}
.box {
width: 33.333%;
}
One box will take up 33.333% width of the container. So, only 3 boxes should be present in first row. But if you check the output it looks like below:

We can see that the boxes are shrinking themselves and staying in the first row. To change that we need to change flex-wrap
to wrap
. Then, the output would look something like below:
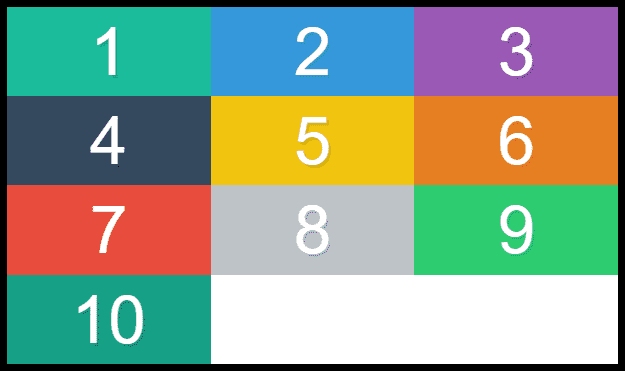
Now, we get the expected result. There is one more value for flex-wrap
which is wrap-reverse
. As expected, it reverses the flex items as seen in the output below:
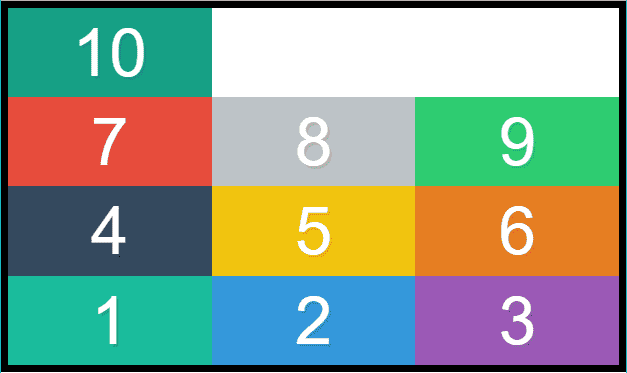
order
In Flexbox, we can change the order or precendence of individual flex items by applying the Flexbox property order
. By default all flex items have order
set to 0
. If we set order
of one box (say box 3) to 1
then it will appear after all the flex items which have order less than 1
.
Let's look an example here:
.box3 {
order: 1;
}

All the boxes except box 3 have order 0
so they appear before box 3. Similarly, if we change the order of box 5 to -1
then it will appear before all the boxes as seen below:
.box5 {
order: -1;
}

Order of flex items is a relative concept. The order of a flex item will depend upon the order of other flex items. For an example if you set the order of box 3 to 100, even then it does not imply that box 3 will appear last in this list. Box 3 will appear last on the list if and only if other boxes have order less than 100.
Once again, I would like to remind that I am taking a free Flexbox course What The Flex Box by Wes Bos. It is a great course and you should check it out if you want to learn Flexbox.